Button
<quiet-button>
Buttons allow users to navigate, submit forms, and perform other actions.
Examples Jump to heading
Variants Jump to heading
Set the variant
attribute to default
, primary
, or
destructive
to change the button's variant.
<quiet-button variant="default"> Default </quiet-button> <quiet-button variant="primary"> Primary </quiet-button> <quiet-button variant="destructive"> Destructive </quiet-button>
There is no constructive
button by design. Use the primary
variant for
constructive actions.
Start & end icons Jump to heading
Use the start
and end
slots to add icons. For best results, use a
<quiet-icon>
or an <svg>
element.
<quiet-button> <quiet-icon slot="start" name="settings"></quiet-icon> Settings </quiet-button> <quiet-button> Favorite <quiet-icon slot="end" name="heart"></quiet-icon> </quiet-button> <quiet-button> <quiet-icon slot="start" name="link"></quiet-icon> Open <quiet-icon slot="end" name="external-link"></quiet-icon> </quiet-button>
Link buttons Jump to heading
Any button can be rendered as a link by setting the href
attribute. This is useful to allow
buttons to act as navigation. When href
is present, other link options such as
download
, rel
, and target
also become available.
<quiet-button href="https://example.com/" target="_blank" rel="noreferrer noopener"> I'm secretly a link </quiet-button> <quiet-button href="/assets/images/wordmark-light.svg" download> Download the logo </quiet-button>
Because link buttons become anchors, they cannot be used with disabled
or
loading
.
Changing the width Jump to heading
To change a button's width, use the CSS width
property.
<quiet-button style="width: 100%"> I'm much longer now </quiet-button>
Changing the size Jump to heading
Use the size
attribute to change the button's size. Available sizes include xs
,
sm
, md
, lg
, and xl
, with the default being
md
.
<quiet-button size="xs">Extra small</quiet-button> <quiet-button size="sm">Small</quiet-button> <quiet-button size="md">Medium</quiet-button> <quiet-button size="lg">Large</quiet-button> <quiet-button size="xl">Extra large</quiet-button>
Outline buttons Jump to heading
Set the appearance
attribute to outline
to draw outlined buttons.
<quiet-button appearance="outline" variant="default"> Default </quiet-button> <quiet-button appearance="outline" variant="primary"> Primary </quiet-button> <quiet-button appearance="outline" variant="destructive"> Destructive </quiet-button>
Text buttons Jump to heading
Set the appearance
attribute to text
to draw text buttons. Text buttons share the
same side and padding as normal buttons, but don't have a background.
<quiet-button appearance="text"> I'm text </quiet-button>
Image buttons Jump to heading
Image buttons are a special appearance that let you create application icons and other picture-based buttons
using images. Set the appearance
attribute to image
to create an image button.
Image buttons are designed to conform to the size of the image you slot in.
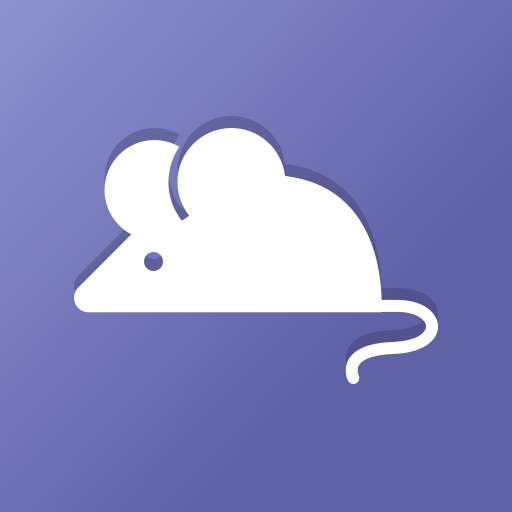
<quiet-button appearance="image"> <img src="/assets/images/app-icon.png" alt="Launch Quiet" style="width: 60px; height: 60px;"> </quiet-button>
Don't forget to include alt
text on images for assistive devices.
Pill-shaped buttons Jump to heading
Buttons can be rendered with pill-shaped edges by adding the pill
attribute.
<quiet-button pill variant="default"> Default </quiet-button> <quiet-button pill variant="primary"> Primary </quiet-button> <quiet-button pill variant="destructive"> Destructive </quiet-button>
Icon buttons Jump to heading
To create an icon button, place an icon into the button's default slot and set the
icon-label
attribute to an appropriate label. The label won't be visible, but it will be
available to assistive devices.
<quiet-button icon-label="Accept" variant="primary"> <quiet-icon name="check"></quiet-icon> </quiet-button> <quiet-button icon-label="Reload"> <quiet-icon name="refresh"></quiet-icon> </quiet-button> <quiet-button icon-label="Delete" variant="destructive"> <quiet-icon name="trash"></quiet-icon> </quiet-button> <quiet-button icon-label="Close" appearance="text"> <quiet-icon name="x"></quiet-icon> </quiet-button>
Toggle buttons Jump to heading
Create a toggle button by adding the toggle="off"
attribute. To make it active by
default, use toggle="on"
instead. An indicator is shown to make it obvious when the
toggle is selected.
<quiet-button variant="default" toggle="off" icon-label="Filter"> <quiet-icon name="filter"></quiet-icon> </quiet-button> <quiet-button variant="primary" toggle="off" icon-label="Alarm"> <quiet-icon name="bell"></quiet-icon> </quiet-button> <quiet-button variant="destructive" toggle="off" icon-label="Record"> <quiet-icon name="microphone"></quiet-icon> </quiet-button> <quiet-button appearance="text" toggle="off" icon-label="Mute"> <quiet-icon name="volume-3"></quiet-icon> </quiet-button>
Toggle buttons cannot be used with link buttons or submit buttons.
Loading buttons Jump to heading
To show the button in a loading state, add the loading
attribute. When a button is loading, it
is effectively disabled and click
events will not be emitted.
<quiet-button loading> I'm loading </quiet-button>
Disabling Jump to heading
To disable a button, add the disabled
attribute. When a button is disabled, the
click
event will not be emitted.
<quiet-button disabled>Regular button</quiet-button> <quiet-button href="https://example.com/" disabled>Link button</quiet-button>
Adding a dropdown caret Jump to heading
A caret can be shown to indicate the button opens a dropdown menu by adding the
with-caret
attribute.
<quiet-button with-caret>Dropdown</quiet-button>
Listening for long press Jump to heading
You can detect long presses by listening to the quiet-long-press
event. The event is dispatched
when the button is clicked or tapped and held for 700 milliseconds.
You can determine if the initiating event was a click (pointer
) or tap (touch
) by
looking at event.detail.type
. You can also look at event.detail.originalEvent
to
get the coordinates and other details.
<quiet-button id="button__long-press">Press and hold</quiet-button> <script> const button = document.getElementById('button__long-press'); button.addEventListener('quiet-long-press', async event => { // Log the coordinates and the type of event that initiated the // long press, i.e. pointer (mouse) or touch (tap) const type = event.detail.type; const { clientX, clientY } = event.detail.originalEvent; console.log(`${type} at ${parseInt(clientX)}×${parseInt(clientY)}`); // Animate the button button.animate([ { scale: 1, offset: 0 }, { scale: 1.2, offset: 0.1 }, { scale: 1, offset: 1 } ], { duration: 500, easing: 'linear' }); }); </script>
API Jump to heading
Importing Jump to heading
The autoloader is the recommended way to import components but, if you prefer to do it manually, the following code snippets will be helpful.
To manually import <quiet-button>
from the CDN, use the following code.
import 'https://cdn.jsdelivr.net/npm/@quietui/quiet-browser@1.0.0/dist/components/button/button.js';
To manually import <quiet-button>
from npm, use the following code.
import '@quietui/quiet/dist/components/button/button.js';
Slots Jump to heading
Button supports the following slots. Learn more about using slots
Name | Description |
---|---|
(default) | The button's label. |
start
|
An icon or similar element to place before the label. Works great with
<quiet-icon> .
|
end
|
An icon or similar element to place after the label. Works great with
<quiet-icon> .
|
Properties Jump to heading
Button has the following properties that can be set with corresponding attributes. In many cases, the attribute's name is the same as the property's name. If an attribute is different, it will be displayed after the property. Learn more about attributes and properties
Property / Attribute | Description | Reflects | Type | Default |
---|---|---|---|---|
appearance
|
Determines the button's appearance. |
|
'normal'
|
'normal'
|
variant
|
The type of button to render. This attribute has no effect on text or image buttons. |
|
'default'
|
'default'
|
disabled
|
Disables the button. |
|
boolean
|
false
|
loading
|
Draws the button in a loading state. |
|
boolean
|
false
|
toggle
|
Turns the button into a two-state toggle button. Clicking once will turn it on. Clicking again will turn it off. Cannot be used with links buttons or submit buttons. |
|
'on'
|
|
size
|
The button's size. |
|
'xs'
|
'md'
|
iconLabel
icon-label
|
To create an icon button, slot an icon into the button's default slot and set this attribute to an appropriate label. The label won't be visible, but it will be available to assistive devices. |
|
string
|
|
pill
|
Draws the button in a pill shape. |
|
boolean
|
false
|
type
|
Determines the button's type. |
|
'button'
|
'button'
|
name
|
The name to submit when the button is used to submit the form. |
|
string
|
|
value
|
The value to submit when the button is used to submit the form. |
|
string
|
''
|
withCaret
with-caret
|
When true, the button will be rendered with a caret to indicate a dropdown menu. |
|
boolean
|
false
|
href
|
Set this to render the button as an <a> tag instead of a
<button> . The button will act as a link. When this is set, form attributes and
features will not work.
|
|
string
|
|
target
|
Opens the link in the specified target. Only works with link buttons. |
|
'_blank'
|
|
rel
|
Sets the link's rel attribute. Only works with link buttons. When linking to an
external domain, you should probably set this to noreferrer noopener .
|
|
string
|
|
download
|
Sets the link's download attribute, causing the linked file to be downloaded. Only
works with link buttons.
|
|
string
|
|
form
|
The form to associate this control with. If omitted, the closest containing
<form> will be used. The value of this attribute must be an ID of a form in the
same document or shadow root.
|
|
string
|
|
formAction
formaction
|
Overrides the containing form's action attribute. |
|
string
|
|
formEnctype
formenctype
|
Overrides the containing form's enctype attribute. |
|
'application/x-www-form-urlencoded'
|
|
formMethod
formmethod
|
Overrides the containing form's method attribute. |
|
'post'
|
|
formNoValidate
formnovalidate
|
Overrides the containing form's novalidate attribute. |
|
boolean
|
|
formTarget
formtarget
|
Overrides the containing form's target attribute. |
|
'_self'
|
Events Jump to heading
Button dispatches the following custom events. You can listen to them the same way was native events. Learn more about custom events
Name | Description |
---|---|
quiet-blur |
Emitted when the button loses focus. This event does not bubble. |
quiet-long-press |
Emitted when the button is pressed and held by tapping or with the mouse. You can look at
event.detail.originalEvent.type to see the type of event that initiated the long press.
|
quiet-focus |
Emitted when the button receives focus. This event does not bubble. |
CSS parts Jump to heading
Button exposes internal elements that can be styled with CSS using the selectors shown below. Learn more about CSS parts
Name | Description | CSS selector |
---|---|---|
button |
The internal <button> element. Other than width , this is where most
custom styles should be applied.
|
::part(button)
|
caret |
The caret icon, a <quiet-icon> element. Only present with the
with-caret attribute.
|
::part(caret)
|
caret__svg |
The caret icon's <svg> part. |
::part(caret__svg)
|
spinner |
The loading indicator, a <quiet-spinner> element. Only present with the
loading attribute.
|
::part(spinner)
|
toggle-indicator |
When the button is a toggle button, this is the indicator that shows the current state. |
::part(toggle-indicator)
|
Custom States Jump to heading
Button has the following custom states. You can target them with CSS using the selectors shown below. Learn more about custom states
Name | Description | CSS selector |
---|---|---|
disabled |
Applied when the button is disabled. |
:state(disabled)
|
focused |
Applied when the button has focus. |
:state(focused)
|
loading |
Applied when a toggle button is loading. |
:state(loading)
|
toggled |
Applied when a toggle button is activated. |
:state(toggled)
|
Dependencies Jump to heading
Button automatically imports the following elements. Sub-dependencies are also included in this list.