Copy
<quiet-copy>
Copy buttons let you copy text and other types of data to the clipboard.
<quiet-copy data="https://quietui.org/"></quiet-copy>
This component uses the Clipboard API's
writeText()
method, which requires a
secure context. In other words, for maximum browser support, make sure the URL starts with https://
.
Examples Jump to heading
Copying text Jump to heading
Use the data
attribute to set the text that will be copied when the button is activated.
<quiet-copy data="Easy as 1-2-3"></quiet-copy>
Using custom buttons Jump to heading
Use a custom button by simply slotting it in. Both Quiet buttons and native buttons are supported.
<quiet-copy data="Copied with a Quiet button" style="margin-inline-end: 0.5rem;"> <quiet-button> <quiet-icon slot="start" name="clipboard"></quiet-icon> Copy </quiet-button> </quiet-copy> <quiet-copy data="Copied with a native button"> <button>Copy</button> </quiet-copy>
Feedback placement Jump to heading
Copy feedback shows above the button by default. Use the feedback-placement
attribute to change
where it gets shown. The actual placement may vary if there's insufficient room to display it in the
viewport. To hide the feedback, set it to hidden
.
<quiet-copy data="Top feedback"></quiet-copy> <quiet-copy feedback-placement="bottom" data="Bottom feedback"></quiet-copy> <quiet-copy feedback-placement="left" data="Left feedback"></quiet-copy> <quiet-copy feedback-placement="right" data="Right feedback"></quiet-copy>
Copying HTML content Jump to heading
You can copy HTML content using the
ClipboardItem API. For best results, make sure to provide text/html
and text/plain
types.
This content will be copied to the clipboard. Try pasting it into another app.
<p> This content will be copied to the <a href="https://en.wikipedia.org/wiki/Clipboard_(computing)" target="_blank">clipboard</a>. <em>Try pasting it into another app.</em> </p> <quiet-copy id="copy__html"> <quiet-button>Copy HTML</quiet-button> </quiet-copy> <script> const copyButton = document.getElementById('copy__html'); const el = copyButton.previousElementSibling; if (window.ClipboardItem) { copyButton.data = [ new ClipboardItem({ 'text/plain': new Blob([el.outerHTML], { type: 'text/plain' }), 'text/html': new Blob([el.outerHTML], { type: 'text/html' }) }) ]; } copyButton.addEventListener('quiet-copy-error', event => { event.preventDefault(); alert(`Sorry, but your browser doesn't support this API yet.`); }); </script>
Copying PNG images Jump to heading
Most browsers support writing PNG image data with the ClipboardItem
API used in this example.
Try copying the image using the button below, then paste it into an image editing app.
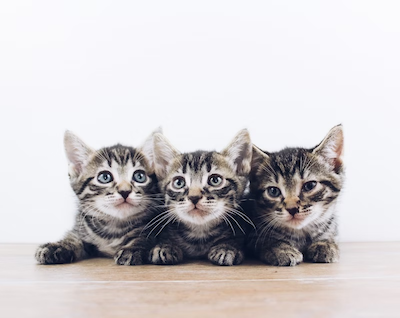
<img src="/assets/examples/three-kittens.png" alt="Three tabby kittens" style="width: 200px; margin-block-end: 1rem;"> <quiet-copy id="copy__png"> <quiet-button>Copy PNG image</quiet-button> </quiet-copy> <script> const copyButton = document.getElementById('copy__png'); if (window.ClipboardItem) { fetch('/assets/examples/three-kittens.png') .then(res => res.blob()) .then(blob => { copyButton.data = [new ClipboardItem({ 'image/png': blob })]; }); } copyButton.addEventListener('quiet-copy-error', event => { event.preventDefault(); alert(`Sorry, but your browser doesn't support this API yet.`); }); </script>
Copying non-PNG images Jump to heading
Copying non-PNG images a trickier due to limited browser support. You can use web custom formats, but they don't seem to be widely supported by platform applications yet.
For now, the most effective way to copy a non-PNG image is to render it in a
<canvas>
element, convert it to a blob, and copy it as a PNG.
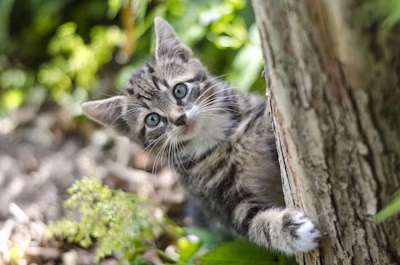
<img src="/assets/examples/kitten-behind-tree.jpeg" alt="A kitten posing behind a tree" style="width: 200px; margin-block-end: 1rem;"> <quiet-copy id="copy__other"> <quiet-button>Copy JPEG image</quiet-button> </quiet-copy> <script> function setCopyDataFromCanvas(image) { // Render the image in a canvas const canvas = document.createElement('canvas'); const ctx = canvas.getContext('2d'); canvas.width = image.naturalWidth; canvas.height = image.naturalHeight; ctx.drawImage(image, 0, 0); // Convert the image data to a blob canvas.toBlob(blob => { copyButton.data = [new ClipboardItem({ 'image/png': blob })]; }); } const copyButton = document.getElementById('copy__other'); if (window.ClipboardItem) { // Load the image to copy const image = new Image(); image.src = '/assets/examples/kitten-behind-tree.jpeg'; image.addEventListener('load', () => setCopyDataFromCanvas(image)); } copyButton.addEventListener('quiet-copy-error', event => { event.preventDefault(); alert(`Sorry, but your browser doesn't support this API yet.`); }); </script>
Disabling Jump to heading
Add the disabled
attribute to disable the button and prevent copying.
<quiet-copy data="https://quietui.org/" disabled></quiet-copy>
API Jump to heading
Importing Jump to heading
The autoloader is the recommended way to import components but, if you prefer to do it manually, the following code snippets will be helpful.
To manually import <quiet-copy>
from the CDN, use the following code.
import 'https://cdn.jsdelivr.net/npm/@quietui/quiet@1.0.0/dist/components/copy/copy.js';
To manually import <quiet-copy>
from npm, use the following code.
import '@quietui/quiet/dist/components/copy/copy.js';
Slots Jump to heading
Copy supports the following slots. Learn more about using slots
Name | Description |
---|---|
(default) | A custom button to use instead of the default. |
Properties Jump to heading
Copy has the following properties that can be set with corresponding attributes. In many cases, the attribute's name is the same as the property's name. If an attribute is different, it will be displayed after the property. Learn more about attributes and properties
Property / Attribute | Description | Reflects | Type | Default |
---|---|---|---|---|
data
|
The text content that will be copied to the clipboard. |
|
string
|
''
|
disabled
|
Disables the button. |
|
boolean
|
false
|
feedbackPlacement
feedback-placement
|
The placement of the feedback animation. |
|
'top'
|
'top'
|
Events Jump to heading
Copy dispatches the following custom events. You can listen to them the same way was native events. Learn more about custom events
Name | Description |
---|---|
quiet-copied |
Emitted when the content has been copied. This event does not bubble. You can inspect
event.detail.data to see the content that was copied.
|
quiet-copy-error |
Emitted when the browser refuses to allow the content to be copied. This event does not bubble. You
can inspect event.detail.error to see the error that occurred.
|
CSS parts Jump to heading
Copy exposes internal elements that can be styled with CSS using the selectors shown below. Learn more about CSS parts
Name | Description | CSS selector |
---|---|---|
copy-button |
The default copy button, a <quiet-button> element.
|
::part(copy-button)
|
copy-button__button |
The default copy button's exported button part. |
::part(copy-button__button)
|
copy-icon |
The default copy icon, a <quiet-icon> element. |
::part(copy-icon)
|
copy-icon__svg |
The copy icon's svg part. |
::part(copy-icon__svg)
|
feedback |
The feedback that shows when copying. |
::part(feedback)
|
Dependencies Jump to heading
Copy automatically imports the following elements. Sub-dependencies are also included in this list.